Grove - Button
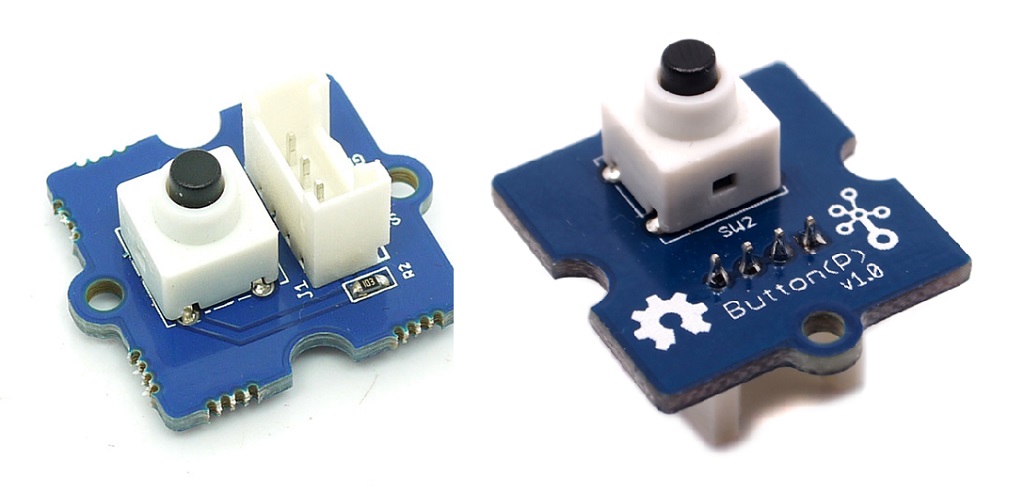
Grove - Button is a momentary push button. It contains one independent "momentary on/off" button. “Momentary” means that the button rebounds on its own after it is released. The button outputs a HIGH signal when pressed, and LOW when released. The Sig marked on silk layer stands for signal while NC stands for not used at all. There are two versions of this button available as showed in the pictures. The only difference is the direction of the Grove socket.
Version
Product Version | Changes | Released Date |
---|---|---|
Grove-Button | Initial | Nov 25 2010 |
Features
- Easy to use momentary ON/OFF button
- Uses Standard 4-pin Grove Cables
More details about Grove modules please refer to Grove System
Specification
Parameter | Value/Range |
---|---|
Operating voltage | 3.3/5V |
Electrical Life | 200,000 cycles |
Operation Force | 100 ± 50gf |
Operation Temperature | -25℃ to +70℃ |
Size | 20mmX20mm |
Platforms Supported
Arduino | Raspberry Pi | |||
---|---|---|---|---|
![]() | ![]() | ![]() | ![]() | ![]() |
The platforms mentioned above as supported is/are an indication of the module's software or theoritical compatibility. We only provide software library or code examples for Arduino platform in most cases. It is not possible to provide software library / demo code for all possible MCU platforms. Hence, users have to write their own software library.
Getting Started
Play With Arduino
Hardware
- Step 1. Prepare the below stuffs:
Seeeduino V4.2 | Base Shield | Grove - Button |
---|---|---|
![]() | ![]() | ![]() |
Get ONE Now | Get ONE Now | Get ONE Now |
- Step 2. Connect Grove-Button to port D2 of Grove-Base Shield.
- Step 3. Plug Grove - Base Shield into Seeeduino.
- Step 4. Connect Seeeduino to PC through a USB cable.
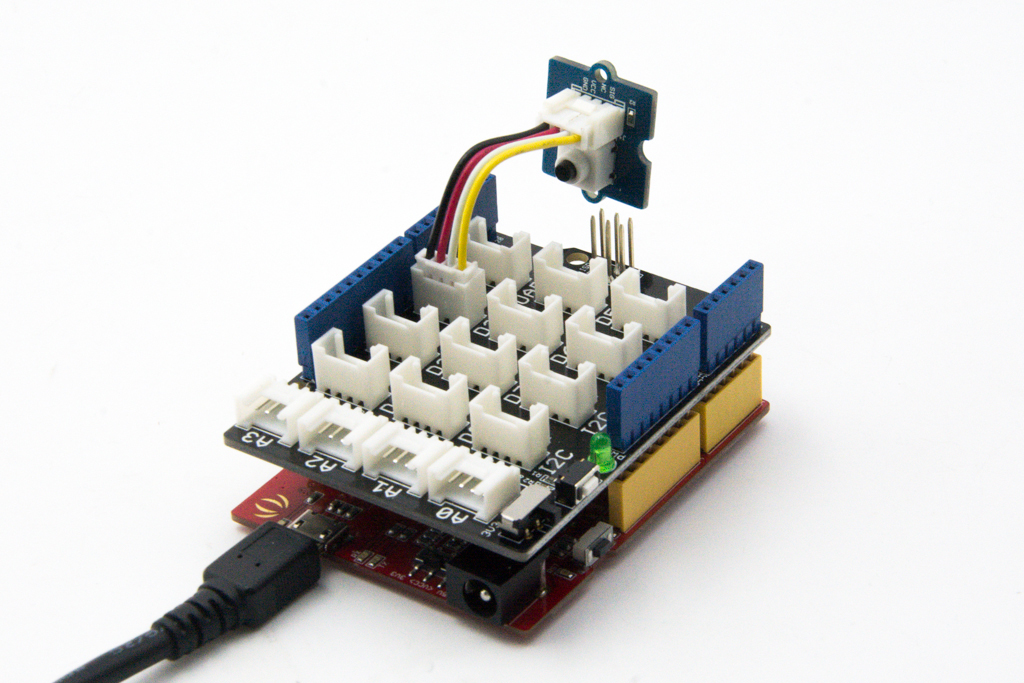
If we don't have Grove Base Shield, We also can directly connect Grove-Button to Seeeduino as below.
Seeeduino | Grove-Button |
---|---|
5V | Red |
GND | Black |
Not Conencted | White |
D2 | Yellow |
Software
- Step 1. Copy the code into Arduino IDE and upload.
const int buttonPin = 2; // the number of the pushbutton pin
const int ledPin = 13; // the number of the LED pin
// variables will change:
int buttonState = 0; // variable for reading the pushbutton status
void setup() {
// initialize the LED pin as an output:
pinMode(ledPin, OUTPUT);
// initialize the pushbutton pin as an input:
pinMode(buttonPin, INPUT);
}
void loop(){
// read the state of the pushbutton value:
buttonState = digitalRead(buttonPin);
// check if the pushbutton is pressed.
// if it is, the buttonState is HIGH:
if (buttonState == HIGH) {
// turn LED on:
digitalWrite(ledPin, HIGH);
}
else {
// turn LED off:
digitalWrite(ledPin, LOW);
}
}
- Step 2. We will see the on board Pin13 LED on and off.
Play with Codecraft
Hardware
Step 1. Connect a Grove - Button to port D2 of a Base Shield.
Step 2. Plug the Base Shield to your Seeeduino/Arduino.
Step 3. Link Seeeduino/Arduino to your PC via an USB cable.
Software
Step 1. Open Codecraft, add Arduino support, and drag a main procedure to working area.
If this is your first time using Codecraft, see also Guide for Codecraft using Arduino.
Step 2. Drag blocks as picture below or open the cdc file which can be downloaded at the end of this page.
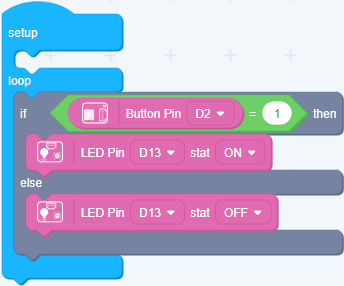
Upload the program to your Arduino/Seeeduino.
tipsuccess When the code finishes uploaded, the LED on the Arduino/Seeeduino board will goes on when the Button pressed.
Play With Raspberry Pi (With Grove Base Hat for Raspberry Pi)
Hardware
- Step 1. Things used in this project:
Raspberry pi | Grove Base Hat for RasPi | Grove - Button |
---|---|---|
![]() | ![]() | ![]() |
Get ONE Now | Get ONE Now | Get ONE Now |
- Step 2. Plug the Grove Base Hat into Raspberry Pi.
- Step 3. Connect the Grove - Button to the PWM port(port 12) of the Base Hat.
- Step 4. Connect the Raspberry Pi to PC through USB cable.
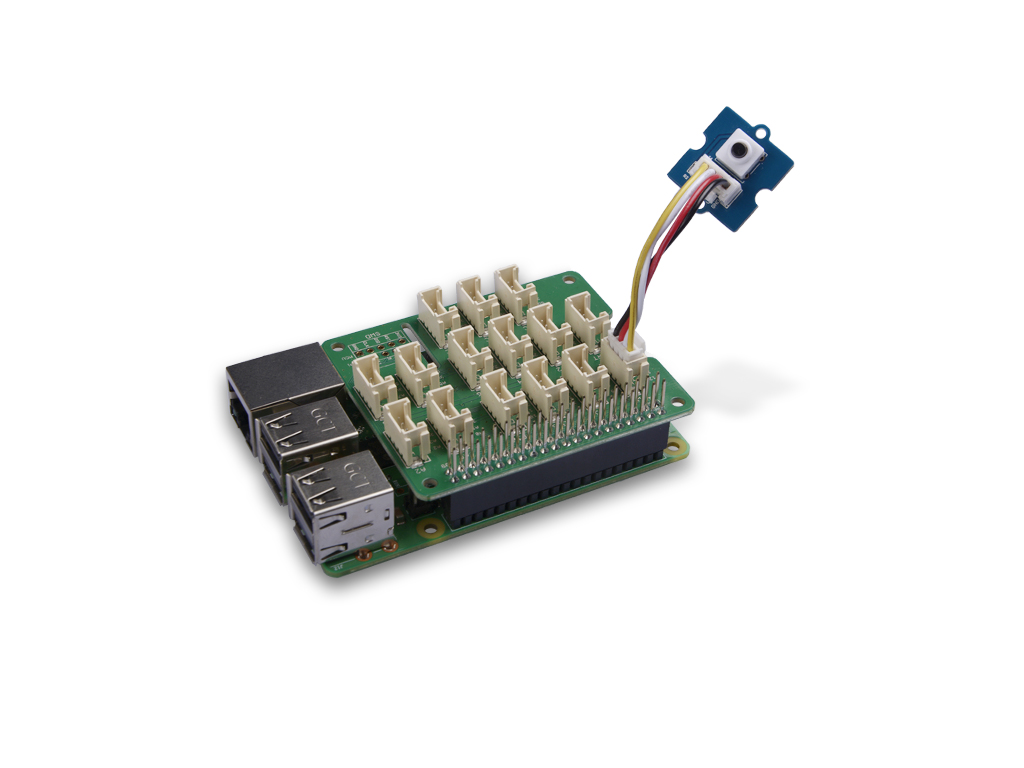
Software
If you are using Raspberry Pi with Raspberrypi OS >= Bullseye, you have to use this command line only with Python3.
- Step 1. Follow Setting Software to configure the development environment.
- Step 2. Download the source file by cloning the grove.py library.
cd ~
git clone https://github.com/Seeed-Studio/grove.py
- Step 3. Excute below command to run the code.
cd grove.py/grove
python3 grove_button.py 12
If you connect the Red LED to the different port of the Base Hat, instead of excuting python grove_led.py 12, you should run the following command.
python3 grove_button.py portnumber
Following is the grove_button.py code.
import time
from grove.button import Button
from grove.factory import Factory
class GroveButton(object):
def __init__(self, pin):
# High = pressed
self.__btn = Factory.getButton("GPIO-HIGH", pin)
self.__last_time = time.time()
self.__on_press = None
self.__on_release = None
self.__btn.on_event(self, GroveButton.__handle_event)
@property
def on_press(self):
return self.__on_press
@on_press.setter
def on_press(self, callback):
if not callable(callback):
return
self.__on_press = callback
@property
def on_release(self):
return self.__on_release
@on_release.setter
def on_release(self, callback):
if not callable(callback):
return
self.__on_release = callback
def __handle_event(self, evt):
dt, self.__last_time = evt["time"] - self.__last_time, evt["time"]
# print("event index:{} event:{} pressed:{}".format(evt["index"], evt["code"], evt["pressed"]))
if evt["code"] == Button.EV_LEVEL_CHANGED:
if evt["pressed"]:
if callable(self.__on_press):
self.__on_press(dt)
else:
if callable(self.__on_release):
self.__on_release(dt)
Grove = GroveButton
def main():
from grove.helper import SlotHelper
sh = SlotHelper(SlotHelper.GPIO)
pin = sh.argv2pin()
button = GroveButton(pin)
def on_press(t):
print('Button is pressed')
def on_release(t):
print("Button is released, pressed for {0} seconds".format(round(t,6)))
button.on_press = on_press
button.on_release = on_release
while True:
time.sleep(1)
if __name__ == '__main__':
main()
If everything goes well, you will be able to see the following result:
pi@raspberrypi:~/grove.py/grove $ python3 grove_button.py 12
Hat Name = 'Grove Base Hat RPi'
Button is pressed
Button is pressed
Button is pressed
Button is pressed
Button is pressed
Button is pressed
Button is released, pressed for 0.002685 seconds
Button is pressed
Button is released, pressed for 0.219019 seconds
Button is pressed
Button is released, pressed for 0.001372 seconds
Button is pressed
Button is pressed
Button is released, pressed for 0.043143 seconds
Button is pressed
Button is released, pressed for 1.083292 seconds
^CTraceback (most recent call last):
File "grove_button.py", line 103, in <module>
main()
File "grove_button.py", line 99, in main
time.sleep(1)
KeyboardInterrupt
You can press ctrl
+c
to quit this program.
Play With Raspberry Pi(with GrovePi_Plus)
Hardware
- Step 1. Prepare the below stuffs:
Raspberry pi | GrovePi_Plus | Grove - Button |
---|---|---|
![]() | ![]() | ![]() |
Get ONE Now | Get ONE Now | Get ONE Now |
- Step 2. Plug the GrovePi_Plus into Raspberry.
- Step 3. Connect Grove-Button to D3 port of GrovePi_Plus.
- Step 4. Connect the Raspberry to PC through USB cable.
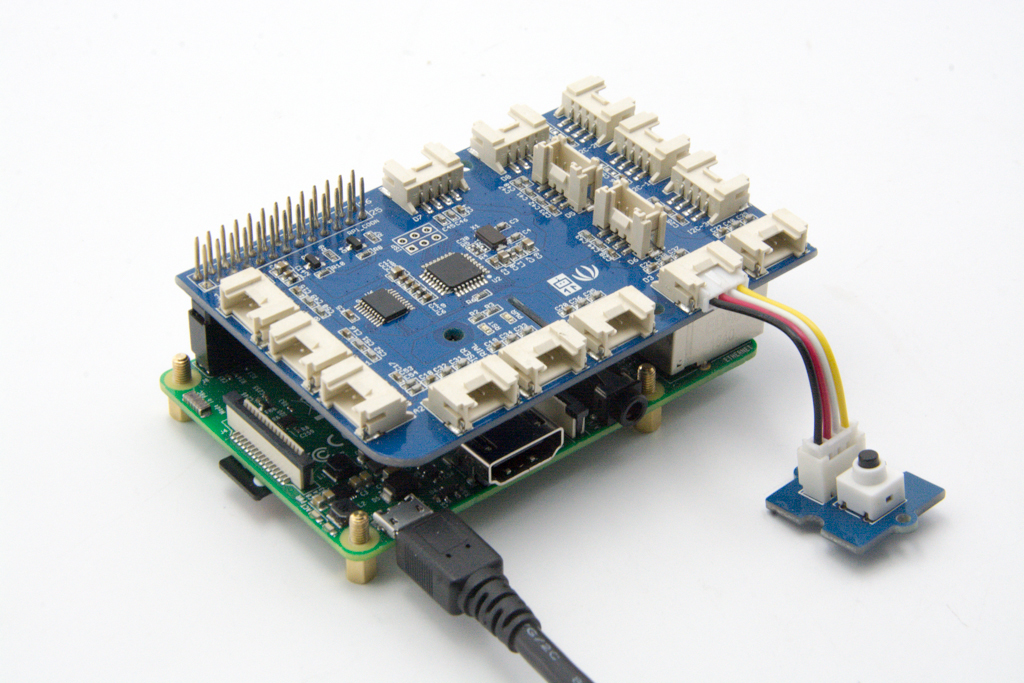
Software
If you are using Raspberry Pi with Raspberrypi OS >= Bullseye, you have to use this command line only with Python3.
- Step 1. Follow Setting Software to configure the development environment.
- Step 2. Git clone the Github repository.
cd ~
git clone https://github.com/DexterInd/GrovePi.git
- Step 3. Excute below commands.
cd ~/GrovePi/Software/Python
python3 grove_button.py
Here is the grove_button.py code.
import time
import grovepi
# Connect the Grove Button to digital port D3
# SIG,NC,VCC,GND
button = 3
grovepi.pinMode(button,"INPUT")
while True:
try:
print(grovepi.digitalRead(button))
time.sleep(.5)
except IOError:
print ("Error")
- Step 4. We will see the button on and off.
pi@raspberrypi:~/GrovePi/Software/Python $ python3 grove_button.py
0
1
1
1
1
0
0
Schematic Online Viewer
Resources
[Eagle&PDF] Grove-Button Eagle Files
[More Reading] Wooden Laser Gun
[Codecraft] CDC File
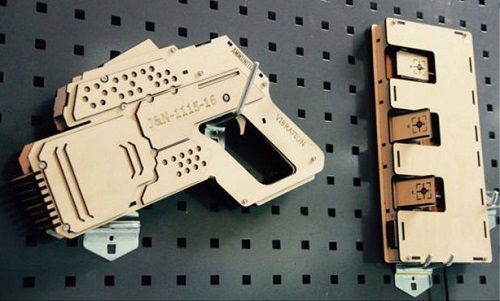
Inspired by OVERWATCH, we have made a very cool Wooden Laser Gun toy for fun these day!
The Wooden Laser Gun and the Gun Target are all based on an Arduino board called Seeeduino Lotus. The laser emitter on the Laser Gun is controlled to fire laser pulse to "activate" the Gun Target. And there are 3 light sensors on the Gun Target to detect the laser pulse. It seems very simple right? If you are interested in our project, please make one for yourself or your child! It's worth to spend one day DIY it as a Xmas present.
Projects
Grove - Introduction in a Button & LED String Light: Beginner-Example - I bet Beginners will smile after project - sent me an selfie!
Using Grove Button To Control Grove LED: How to connect and use Grove Button to control Grove LED socket kit.
Button and LED Grove modules:
Tech Support & Product Discussion
Thank you for choosing our products! We are here to provide you with different support to ensure that your experience with our products is as smooth as possible. We offer several communication channels to cater to different preferences and needs.